Read Also: Collection Interview Questions
To convert HashMap to ArrayList we need to convert HashMap keys and HashMap values to ArrayList. Alternatively, we can convert key-value pairs into ArrayList. In this article, we will learn several methods to perform the above conversions with or without using Java 8 Stream API. Let's dive deep into the topic.
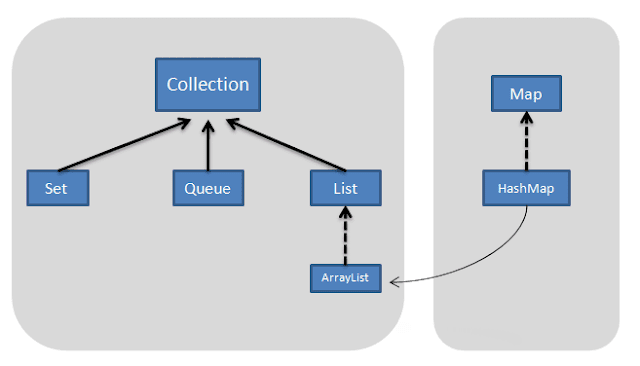
Convert HashMap to ArrayList using Java 8
In Java 8, we can use below two methods to convert HashMap into ArrayList:1. Conversion of HashMap Keys to ArrayList
import java.util.*; import java.util.Map.Entry; import java.util.stream.Collectors; public class JavaHungry { public static void main(String args[]) { //Creating a HashMap Object HashMap<Integer,Integer> map = new HashMap<>(); //Adding Values to HashMap map.put(1,10); map.put(2,20); map.put(3,30); map.put(4,40); map.put(5,50); //Creating an ArrayList Object and adding HashMap keys to it using Java 8 ArrayList<Integer> list = (ArrayList<Integer>)map.keySet().stream().collect(Collectors.toList()); System.out.println("ArrayList of Keys: "); //Displaying the contents of ArrayList of keys in Java 8 list.forEach(System.out::println); } }
Output:
ArrayList of Keys:
1
2
3
4
5
2. Conversion of HashMap Values to ArrayList
import java.util.*; import java.util.Map.Entry; import java.util.stream.Collectors; public class JavaHungry { public static void main(String args[]) { //Creating a HashMap Object HashMap<Integer,Integer> map = new HashMap<>(); //Adding Values to HashMap map.put(1,10); map.put(2,20); map.put(3,30); map.put(4,40); map.put(5,50); //Creating an ArrayList Object and adding HashMap items to it using Java 8 ArrayList<Integer> list = (ArrayList<Integer>)map.values().stream().collect(Collectors.toList()); System.out.println("ArrayList of Values: "); //Displaying the contents of ArrayList of Values in Java 8 list.forEach(System.out::println); } }
Output:
ArrayList of Values:
10
20
30
40
50
Convert HashMap to ArrayList Without Using Stream API
1. Conversion of HashMap Keys into ArrayListIn this method, we will create one ArrayList and add all the keys into it using the keySet() method of HashMap.
import java.util.*; public class JavaHungry { public static void main(String args[]) { //Creating a HashMap Object HashMap<Integer,Integer> map = new HashMap<>(); //Adding Values to HashMap map.put(1,10); map.put(2,20); map.put(3,30); map.put(4,40); map.put(5,50); //Getting Set of Keys from HashMap Set<Integer> keyset = map.keySet(); //Creating an ArrayList Object ArrayList<Integer> list = new ArrayList<>(); //Adding each key to ArrayList for(int key: keyset) { list.add(key); } //Display the content of ArrayList System.out.println("ArrayList of Keys:"); for(int data: list) { System.out.print(data+" "); } } }
Output:
ArrayList of Keys:
1 2 3 4 5
2. Conversion of HashMap Values into ArrayList
In this method, we will create one ArrayList and add all the values into it using values() method of HashMap.
import java.util.*; public class JavaHungry { public static void main(String args[]) { //Creating a HashMap Object HashMap<Integer,Integer> map = new HashMap<>(); //Adding Values to HashMap map.put(1,10); map.put(2,20); map.put(3,30); map.put(4,40); map.put(5,50); //Getting Collection of Values from HashMap Collection<Integer> val = map.values(); //Creating an ArrayList Object ArrayList<Integer> list = new ArrayList<>(); //Adding each Value to ArrayList for(int value: val) { list.add(value); } //Display the content of ArrayList System.out.println("ArrayList of Values:"); for(int data : list) { System.out.print(data+" "); } } }
Output:
ArrayList of Values:
10 20 30 40 50
3. Conversion of Key-Value Pairs into ArrayList
For this, we are going to use the entrySet() method of HashMap class. This method returns the set of entries containing key-value pairs i.e. Entry
import java.util.*; import java.util.Map.Entry; public class JavaHungry { public static void main(String args[]) { //Creating a HashMap Object HashMap<Integer,Integer> map = new HashMap<>(); //Adding Values to HashMap map.put(1,10); map.put(2,20); map.put(3,30); map.put(4,40); map.put(5,50); //Getting Set of Entry<Key,Value> from HashMap Set<Entry<Integer,Integer>> entryset = map.entrySet(); //Creating an ArrayList Object ArrayList<Entry<Integer,Integer>> list = new ArrayList<>(); //Adding each Value to ArrayList for(Entry<Integer,Integer> entry: entryset) { list.add(entry); } //Display the content of ArrayList System.out.println("ArrayList of Key-Value Pairs:"); for(Entry<Integer,Integer> data : list) { System.out.print(data+" "); } } }
Output:
ArrayList of Key-Value Pairs:
1=10 2=20 3=30 4=40 5=50
That's all for today, using the above methods, we can convert HashMap to ArrayList by using any current version of Java. Please mention in the comments in case you know any other way.
References:
HashMap Java doc
ArrayList Java doc