Difference between Collection and Collections is an important interview question for java freshers.
This question has been used to test the knowledge of Java Collections Framework. Make sure you go through this question before appearing for the interview.
This question is quite confusing as both Collection and Collections are looking similar by name. But they are completely different. Collection is an interface while Collections is a utility class. In this post, I will be sharing the difference between Collection and Collections in java along with examples.
Read Also : Java Collections Interview Questions and Answers
Collections is a utility class in java which contains only static methods that operate on or return collections.
2. Static methods : Collection is an interface. Interface can contain static methods since java 8. Before java8, interface was not allowed to contain static methods. Interface can also contain abstract methods and default methods.
On the other hand, Collections class contains only static methods.
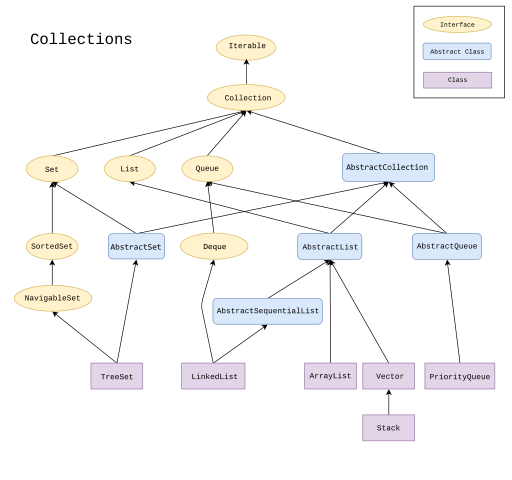
3. extends : Collection interface extends iterable interface.
On the other hand, Collections class extends Object class.
2. Set, Queue and List are some of the main subinterfaces of Collection interface.
3. Map interface is also part of the Collections Framework. But it doesn't inherit Collection interface.
4. The jdk does not provide direct implementation of Collection interface. Although, it provides implementation of specific subinterfaces like Set, List and Queue.
5. HashSet, ArrayList, LinkedHashSet, Vector, PriorityQueue are some indirect implementations of Collection Interface.
6. Collections is a utility class in java which contains only static methods. Using this class methods you can search for a particular element in a collection. It also contains the methods to sort the collection.
Output :
Maximum element in the given list : 55
2. Both are present in java.util package.
3. Both are added to jdk in java version 1.2
Collection interface has methods that let you know about how many elements are in the collection (size() , is Empty()) , methods that check whether a given object is in the collection (contains()), methods that add or remove an element from the collection (add(), remove()).
Please mention in the comments in case you have any doubts regarding the difference between Collection and Collections in java.
References :
Stackoverflow
Image :
Ramlmn [CC BY-SA 4.0], via Wikimedia Commons
This question has been used to test the knowledge of Java Collections Framework. Make sure you go through this question before appearing for the interview.
This question is quite confusing as both Collection and Collections are looking similar by name. But they are completely different. Collection is an interface while Collections is a utility class. In this post, I will be sharing the difference between Collection and Collections in java along with examples.
Read Also : Java Collections Interview Questions and Answers
Difference between Collection and Collections in Java :
1. Type : Collection is a root level interface in Java Collection Framework or collection hierarchy.Collections is a utility class in java which contains only static methods that operate on or return collections.
2. Static methods : Collection is an interface. Interface can contain static methods since java 8. Before java8, interface was not allowed to contain static methods. Interface can also contain abstract methods and default methods.
On the other hand, Collections class contains only static methods.
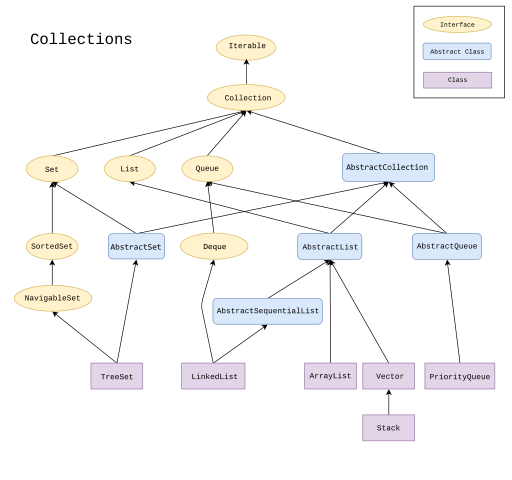
3. extends : Collection interface extends iterable interface.
public interface Collections<E> extends Iterable<E>
On the other hand, Collections class extends Object class.
public class Collections extends Object
Important Points:
1. Most of the java collections framework classes inherit from Collection interface.2. Set, Queue and List are some of the main subinterfaces of Collection interface.
3. Map interface is also part of the Collections Framework. But it doesn't inherit Collection interface.
4. The jdk does not provide direct implementation of Collection interface. Although, it provides implementation of specific subinterfaces like Set, List and Queue.
5. HashSet, ArrayList, LinkedHashSet, Vector, PriorityQueue are some indirect implementations of Collection Interface.
6. Collections is a utility class in java which contains only static methods. Using this class methods you can search for a particular element in a collection. It also contains the methods to sort the collection.
Example of Collections Class
Below is an example of one of the utility methods i.e max() of Collections classimport java.util.*; public class JavaHungry { public static void main(String args[]) { // Creating ArrayList Object ArrayList<Integer> al = new ArrayList<Integer>(); // Adding elements to the ArrayList al.add(10); al.add(45); al.add(55); al.add(23); al.add(32); // max() method of Collections class System.out.println("Maximum element in the given list : "
+ Collections.max(al)); } }
Output :
Maximum element in the given list : 55
Similarities between Collection and Collections in Java
1. Both are part of the Java Collections Framework.2. Both are present in java.util package.
3. Both are added to jdk in java version 1.2
Important Methods in Collections Class
Collections.sort() | sort() method sorts the elements in the given collection. |
Collections.min() | min() method returns the minimum element in the given collection. |
Collections.max() | max() method returns the maximum element in the given collection. |
Collections.binarySearch() | binarySearch() method searches for the specified element in the given collection using binary search algorithm. |
Collections.reverse() | reverse() method returns the reverse order of the elements in the given collection |
Collections.copy() | copy() method copies all elements from one collection to the another collection |
Important Methods in Collection Interface
According to Oracle docs :Collection interface has methods that let you know about how many elements are in the collection (size() , is Empty()) , methods that check whether a given object is in the collection (contains()), methods that add or remove an element from the collection (add(), remove()).
boolean add() |
boolean remove(Object o) |
int size() |
void clear() |
boolean isEmpty() |
Please mention in the comments in case you have any doubts regarding the difference between Collection and Collections in java.
References :
Stackoverflow
Image :
Ramlmn [CC BY-SA 4.0], via Wikimedia Commons